Our genetic makeup, and that of plants and animals, is stored in DNA. DNA is composed of two DNA-Strands, which twist together to form a double helix.
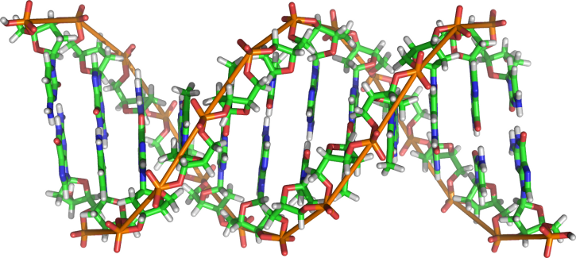
Each strand of DNA is a chain of smaller bases connected in a line. There are four bases:
- adenine (abbreviated A)
- cytosine (C)
- guanine (G)
- thymine (T)
The bases have pairs: A binds to T and C binds to G. The two strands ‘match up’, so that the bases at each point bind to their corresponding pairs. So for example, if one strand was
A-C-G-G-T-C
then the other strand would be:
T-G-C-C-A-G
since we have the pairs A:T, C:G, G:C, G:C, T:A and C:G. This may sound like a redundant way of storing information, but it means that if we pull apart the strands of the double helix and throw one side away, we are still able to reconstruct it. Furthermore, if we want to duplicate our double helix, we can split it into the two strands, reconstruct each side, and then end up with two copies of the original double helix.
In this exercise, we will create a class that represents a strand of DNA.
Here is the API for the class DNAStrand:
- public DNAStrand(String dna)
- Class constructor.
- public boolean isValidDNA()
- Returns true if the DNA is valid, i.e, only contains the letters, A,T,C,G (in uppercase) and at least one of these characters.
- public String complementWC()
- Returns the Watson Crick complement, which is a string representing the complementary DNA strand (i.e., the other strand in the double helix). So swap all T’s with A’s, all A’s with T’s, all C’s with G’s and all G’s with C’s.
- public String palindromeWC()
- Returns the Watson Crick Palindrome, which is the reversed sequence of the complement.
- public boolean containsSequence(String seq)
- Returns true if the DNA contains the subsequence seq.
- public String toString()
- Returns the underlying DNA sequence string.
Write a DNAStrand class which meets this API.
An automated test has been created for this exercise: DNAStrandTest.java.
In addition, create a client class DNAStrandTester which contains the following static method:
public static void summarise(DNAStrand dna) { System.out.println("Original DNA Sequence: " + dna); if (dna.isValidDNA()) { System.out.println("Is valid"); System.out.println("Complement: " + dna.complementWC()); System.out.println("WC Palindrome: " + dna.palindromeWC()); } else { System.out.println("Not Valid DNA"); } }
Create one or more DNAStrand objects inside the main() method of DNAStrandTester and test them with the summarise() method.