The aim of this exercise is to introduce you to some very simple numeric calculations, and the parsing of input data. The task is to create a program called Adder that takes two numbers from the user, sums them and displays the calculated value.
For this process you will probably require variables to store the numbers that have been entered by the user, and to store the result of the sum. Unlike the last exercise where you were asked to store a String, this time you will be storing a simple number. For this, and for the addition to perform correctly you need to use the int (integer) type, as shown below:
int myNumber;
You also need a way to convert the argument that the user provides on the command line from a string into an integer. We will use the method Integer.parseInt(), which parses a String into an int.
Given the code from the previous exercise, we can now read integers from the command line. For example to read the first command line argument as an integer, we would write:
int myNumber = Integer.parseInt(args[0]);
and
int myNumber2 = Integer.parseInt(args[1]);
to read the second one.
The methods System.out.print() and System.out.println() can be used to print out int values as well as Strings.
Write your program to carry out the specified task of summing two integer arguments from the command line. Your session at the terminal should look something like this:
: javac Adder.java : java Adder 1 2 3 : java Adder 3 8 11 : java Adder 34 23 57
Now you should have an idea of how the javac compiler and the java interpreter are being used to translate clear text Java source code into executable binary class files. Also, how to pass in command line arguments when executing the program should be clear.
Let's use this exercise to transition to using IntelliJ for the rest of the course:
- Create a new Java or Gradle project using IntelliJ as explained in the Introduction to Development Tools.
- Copy your Adder.java file into the corresponding source directory of the project using the command line or your operating system's file explorer.
- Execute the main function
You will now likely get a runtime error saying something like this:
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: Index 0 out of bounds for length 0 at Adder.main(Adder.java:4)
This is because you have not provided command line arguments and trying to access them in Adder line 4 fails. To edit command line arguments using IntelliJ, complete the following steps:
- Open the drop down menu next to the run and build button in the top right corner.
- Select Edit Configurations ...
- In the configuration options, fill in your command line arguments under Program Arguments.
- Execute your code again by pressing the arrow in the top right corner or next to your main function.
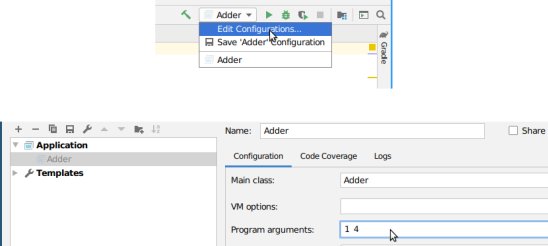
Your program should now execute as intended and calculate the result (if your implementation is correct). If you created a Java project, your output should look similar to this:
/opt/jdk-11.0.4/bin/java -javaagent:/opt/idea-IC-191.7479.19/lib/idea_rt.jar=42025:/opt/idea-IC-191.7479.19/bin -Dfile.encoding=UTF-8 -classpath /home/vseeker/inf1b/labs/Adder/out/production/Adder Adder 1 4 5 Process finished with exit code 0
The initial output shows the command line instruction executed by IntelliJ which is a bit longer than what you did in the previous two exercises. If you are interested, you can use the internet to find out what the additional arguments mean. Note, that in the very end of the first line you should have the usual command line call with the provided arguments Adder 1 4
This initial execution command is followed by the output of your program, i.e. 5.
Lastly, an exit code of zero your executed program process indicates a normal termination without error.
Note
To keep your code organised, we recommend that you create a new IntelliJ project for each individual lab exercise.
An automated test has been created for this exercise: AdderTest.java.
Add it to your IntelliJ project by following the steps explained in the Introduction to Development Tools.